Samples
Description samples for testing.
PTTPluginSample
CryptoPlugin Sample | version 4.3.0 Server 8.0.0~ | Down |
---|---|---|
AdminWebPlugin2 Sample | version 4.2.0 Server 6.3.1~ | Down |
AdminWebPlugin Sample | version 4.1.0 Server 6.3.0~ | Down |
PublicGWPlugin Sample | version 4.0.0 Server 6.1.1~ | Down |
PTTPlugin4 Sample | version 4.0.0 Server 6.0.2~ | Down |
PTTPlugin3 Sample | version 3.0.0 Server 5.1.0~ | Down |
PTTPlugin2.1 Sample | version 2.1.0 Server 5.0.2~ | Down |
PTTPlugin2 Sample | version 2.0.0 Server 3.3.0~ | Down |
PTTPlugin Sample | version 1.0.0 | Down |
PTTPlugin4Sample.java for V4
2FA(Two-Factor Authentication) by email/SMS
Basic flow
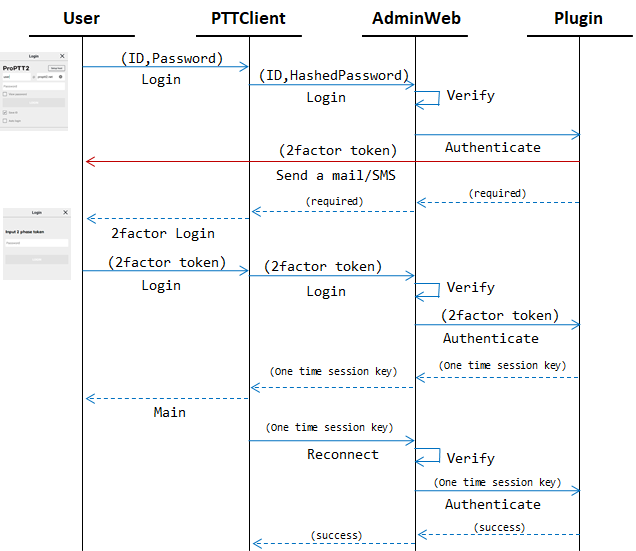
AdminWebPluginSample.java